RandomWay (openGL game)
linux version v1.0
copy the code to a file with the ending ".c"
compile:
gcc name.c -lGL -lGLU -lglut
run:
./a.out
gameplay:
"a" left around
"s" right around
"c" camera 3D
"v" camera 2D
red fields make players slower
green fields make players faster
black fields can't be passed
goal:
find the way to the other obstacle
/*******************************************************************************************************************
SPIEL_1_WPF_v2_0
********************************************************************************************************************/
#include <stdlib.h>
#include <stdio.h>
#include <time.h>
#include <GL/glut.h>
#include <GL/gl.h>
int xSize = 800, ySize = 700; // Screensize for gameplay
/*******************************************************************************************************************
Prototypen OpenGL Callbackfunktionen
********************************************************************************************************************/
void init(void); // Initial conditions
void display(void); // Scene-rendering (OpenGL) Arraypositions to vertices (depending on Drawmode)
void keyboard(unsigned char, int, int); // Input-arguments (Keybord-action)
// void displayViewPort(void);
void idle(void); // time-action for clock tick dependend actions
/********************************************************************************************************************
Init variables and constants (global)
********************************************************************************************************************/
int timebase1;
int x_size, y_size, speed, direction, tick = 0, whole_ticks = 0, xposEnd, yposEnd,camera=0,breite,tiefe, enD = 0;
// Predefinition of used colors in the game
float white[] = {1,1,1,1};
float black[] = {0,0,0,1};
float red[] = {1,0,0,0};
float green[] = {0,1,0,0};
float blue[] = {0,0,1,0};
int xpos, ypos, xcpos, ycpos, hcpos = 10;
// init gamefield-Array (ptr_depth = 2)
int **field = NULL; //0, normales feld
//1, schneller
//2, langsamer,
//3, stop
//immer 5 * 5 felder
/********************************************************************************************************************
Funktiondefinitions for algorithmics
********************************************************************************************************************/
// Cameraposition (Änderung der Laufrichtung <00°,90°,180°,270°>)
void yx_camPos() {
if(direction==1){
xcpos = xpos ;
ycpos = ypos - 15;
}
if(direction==2){
xcpos = xpos + 15;
ycpos = ypos;
}
if(direction==3){
xcpos = xpos ;
ycpos = ypos + 15;
}
if(direction==4){
xcpos = xpos - 15;
ycpos = ypos;
}
}
// generates inital-Gamefield
void create(int x_sizeN, int y_sizeN, int start_xN, int start_yN, int dir, int endposx, int endposy) {
speed = 5; //startgeschwindigkeit 1 bis 10
direction = dir; //1 , nach unten(y achse),
//2 nach links,
//3 nach oben,
//4 nach rechts(pos x achse)
x_size = x_sizeN; //soll modulo 5 = 0 ergeben
y_size = y_sizeN; //soll modulo 5 = 0 ergeben
xpos = start_xN;
ypos = start_yN;
xposEnd = endposx;
yposEnd = endposy;
field = (int**) malloc(x_size * sizeof (int *));
int i, j;
for (i = 0; i < x_size; i++) {
field[i] = (int*) malloc(y_size * sizeof (int));
}
for (i = 0; i < x_size; i++) {
for (j = 0; j < y_size; j++) {
field[i][j] = 0;
}
}
/*//Testausgabe
for(i = 0; i < x_size;i++){
for(j = 0; j < y_size;j++){
field[i][j] = 2 * i * j + 1;
printf("i: %d, j %d, field(i,j)= %d\n",i,j,field[i][j]);
}
}
*/
//return 0;
}
//generates gamefield (erzeugte felder sind immer 5*5 pixel() gross)
int create_field(int nOf_speedup, int nOf_speeddown, int nOf_stop) {
srand(time(NULL));
int i, j, xcor, ycor, x, y;
//langsamer feld
for (i = 0; i < nOf_speeddown; i++) {
xcor = (1+(rand()) % (x_size / 5))*5 - 2;
ycor = (1+(rand()) % (y_size / 5))*5 - 2;
if (field[xcor][ycor] == 0) {
for (x = xcor - 3; x < xcor + 2; x++) {
for (y = ycor - 3; y < ycor + 2; y++) {
field[x][y] = 2;
}
}
} else {
i--;
}
}
//schneller feld
for (i = 0; i < nOf_speedup; i++) {
xcor = (1+(rand()) % (x_size / 5))*5 - 2;
ycor = (1+(rand()) % (y_size / 5))*5 - 2;
if (field[xcor][ycor] == 0) {
for (x = xcor - 3; x < xcor + 2; x++) {
for (y = ycor - 3; y < ycor + 2; y++) {
field[x][y] = 1;
}
}
} else {
i--;
}
}
//stop feld
for (i = 0; i < nOf_stop; i++) {
xcor = (1+(rand()) % (x_size / 5))*5 - 2;
ycor = (1+(rand()) % (y_size / 5))*5 - 2;
if (field[xcor][ycor] == 0) {
for (x = xcor - 3; x < xcor + 2; x++) {
for (y = ycor - 3; y < ycor + 2; y++) {
field[x][y] = 3;
}
}
} else {
i--;
}
}
return 0;
}
// change direction (handling)
void turn(int direction_change) {
//printf("direction = %d ,", direction);
int dir_change = direction_change; // 0 geradeaus, 1 rechts, -1 links
direction += dir_change;
if (direction < 1)
direction += 4;
if (direction > 4)
direction -= 4;
//printf("dir change = %d, dir = %d\n", dir_change,direction);
}
// walkthrew algorithmics <0°,90°,180°,270°> (speedindex depends on fieldarguments)
int tick_method() {
tick++;
whole_ticks++;
if (tick % (11 - speed) == 0) {
tick = 0;
if (direction == 1) {
ypos++;
if (ypos >= y_size) ypos = y_size-1;
if (field[xpos][ypos] == 3) {
ypos--; //if stopfeld ypos--
}
}
if (direction == 2) {
xpos--;
if (xpos <= 0) xpos = 0;
if (field[xpos][ypos] == 3) {
xpos++;
}
}
if (direction == 3) {
ypos--;
if (ypos <= 0) ypos = 0;
if (field[xpos][ypos] == 3) {
ypos++;
}
}
if (direction == 4) {
xpos++;
if (xpos >= x_size) xpos = x_size-1;
if (field[xpos][ypos] == 3) {
xpos--;
}
}
if (field[xpos][ypos] == 1) speed++;
if (field[xpos][ypos] == 2) speed--;
if (speed < 1)speed = 1; //speed nicht unter 1
if (speed > 10)speed = 10; //oder ueber 10
}
if(xpos == xposEnd && ypos == yposEnd) return 1;
//printf("%d\n", whole_ticks);
return 0;
}
/*******************************************************************************************************************
main()-function
********************************************************************************************************************/
int main(int argc, char* argv[]) {
int success = 0;
int breite = 50;
int tiefe = 50;
// generating gamefield
create(breite, tiefe, 0, 15, 4, 49,30);
create_field(15, 15, 25);
while(field[xpos][ypos]==3){
ypos-=5;
}
while(field[xposEnd][yposEnd]==3){
yposEnd+=5;
}
// handling C-arguments and inital variables for glut-Engine Interfaces
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE);
glutInitWindowSize(xSize, ySize);
glutInitWindowPosition(20, 100);
glutCreateWindow("_Spiel_1_WPF226 OPENGL_");
init();
glutIdleFunc(idle);
glutDisplayFunc(display);
glutKeyboardFunc(keyboard);
glutMainLoop();
// deallocation of heapspace
free(field);
return 0;
}
/*******************************************************************************************************************
OpenGL (functions-definition)
********************************************************************************************************************/
void init(void) {
glClearColor(1.0, 1.0, 1.0, 0.0);
timebase1 = glutGet(GLUT_ELAPSED_TIME);
glClearColor(1.0, 1.0, 1.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(45,xSize/ySize,0.1,1000);
// 1) to do: light-source
GLfloat light_position[] = { 0.0, 1.0, 0.0, 1.0 };
GLfloat light_diffuse[] = {0.5, 0.5, 0.5, 0.0};
glShadeModel (GL_SMOOTH);
glLightfv(GL_LIGHT0, GL_POSITION, light_position);
glLightfv(GL_LIGHT0, GL_DIFFUSE, light_diffuse);
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
}
// dislays gamefield (vertice-Mode is QUADS)
void display(void) {
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// 2) to do: cameraviews (field, ego)
if(camera == 0) {
gluLookAt(25,25,-70,25,25,0,0,1,0);
} else if(camera == 1) {
//gluLookAt(breite/2,-tiefe/2,-34,12,10,0,0,1,0);
gluLookAt(xcpos,ycpos,-hcpos,xpos,ypos,0,0,0,-1);
}
//glMatrixMode(GL_MODELVIEW);
//glLoadIdentity();
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT | GL_DOUBLEBUFFER);
glPolygonMode(GL_FRONT_AND_BACK, GL_QUADS);
glEnable(GL_DEPTH_TEST);
glBegin(GL_QUADS);
int i,j,k;
double game_f1[3], game_f2[3],game_f3[3], game_f4[3];
glPushMatrix();
// Punkt links oben
game_f1[0] = (double) -1000;
game_f1[1] = (double) +1000;
game_f1[2] = -1.0;
// Punkt rechts oben
game_f2[0] = (double) +1000;
game_f2[1] = (double) +1000;
game_f2[2] = -1.0;
// Punkt links unten
game_f3[0] = (double) -1000;
game_f3[1] = (double) -1000;
game_f3[2] = -1.0;
// Punkt rechts unten
game_f4[0] = (double) +1000;
game_f4[1] = (double) -1000;
game_f4[2] = -1.0;
glColor3d(0.8,0.8,0.8);
glVertex3d(game_f1[0], game_f1[1], game_f1[2]);
glVertex3d(game_f2[0], game_f2[1], game_f2[2]);
glVertex3d(game_f4[0], game_f4[1], game_f4[2]);
glVertex3d(game_f3[0], game_f3[1], game_f3[2]);
for(i = 0; i < x_size; i++) {
for(j = 0; j < y_size; j++) {
// Punkt links oben
game_f1[0] = (double) i-0.5;
game_f1[1] = (double) j-0.5;
game_f1[2] = 0.0;
// Punkt rechts oben
game_f2[0] = (double) i+0.5;
game_f2[1] = (double) j-0.5;
game_f2[2] = 0.0;
// Punkt links unten
game_f3[0] = (double) i-0.5;
game_f3[1] = (double) j+0.5;
game_f3[2] = 0.0;
// Punkt rechts unten
game_f4[0] = (double) i+0.5;
game_f4[1] = (double) j+0.5;
game_f4[2] = 0.0;
if(field[i][j] == 0) {
glColor3d(1.0,1.0,1.0);
glMaterialfv(GL_FRONT, GL_DIFFUSE, white);
glVertex3d(game_f1[0], game_f1[1], game_f1[2]);
glVertex3d(game_f2[0], game_f2[1], game_f2[2]);
glVertex3d(game_f4[0], game_f4[1], game_f4[2]);
glVertex3d(game_f3[0], game_f3[1], game_f3[2]);
}
if(field[i][j] == 1) {
glColor3d(0.0,1.0,0.0);
glMaterialfv(GL_FRONT, GL_DIFFUSE, green);
glVertex3d(game_f1[0], game_f1[1], game_f1[2]);
glVertex3d(game_f2[0], game_f2[1], game_f2[2]);
glVertex3d(game_f4[0], game_f4[1], game_f4[2]);
glVertex3d(game_f3[0], game_f3[1], game_f3[2]);
}
if(field[i][j] == 2) {
glColor3d(1.0,0.0,0.0);
glMaterialfv(GL_FRONT, GL_DIFFUSE, red);
glVertex3d(game_f1[0], game_f1[1], game_f1[2]);
glVertex3d(game_f2[0], game_f2[1], game_f2[2]);
glVertex3d(game_f4[0], game_f4[1], game_f4[2]);
glVertex3d(game_f3[0], game_f3[1], game_f3[2]);
}
if(field[i][j] == 3) {
glColor3d(0.0,0.0,0.0);
glMaterialfv(GL_FRONT, GL_DIFFUSE, black);
glVertex3d(game_f1[0], game_f1[1], game_f1[2]);
glVertex3d(game_f2[0], game_f2[1], game_f2[2]);
glVertex3d(game_f4[0], game_f4[1], game_f4[2]);
glVertex3d(game_f3[0], game_f3[1], game_f3[2]);
}
}
}
glEnd();
glPopMatrix();
glPushMatrix();
glColor3d(0.0,0.0,1.0);
glTranslatef(xposEnd,yposEnd,0.0);
glRotatef(whole_ticks,0.0,1.0,0.0);
glMaterialfv(GL_FRONT, GL_DIFFUSE, blue);
glutSolidCube(0.7);
glPopMatrix();
glColor3d(0.0,0.0,0.0);
glTranslatef(xpos,ypos,0.0);
glMaterialfv(GL_FRONT, GL_DIFFUSE, blue);
glutSolidCube(0.7);
//gluLookAt(0,0,-14000,0,0,0,0,10000,0);
glutSwapBuffers();
//printf("display ende");
}
//void reshape(int w, int h) {
// glViewport(0, 0, (GLsizei) w, (GLsizei) h);
//}
/*
void displayViewPort() {
int vp[4];
glGetIntegerv(GL_VIEWPORT, vp);
}
*/
// keyboard-IO
void keyboard(unsigned char ch, int int1, int int2){
switch(ch){
case 'a': turn(-1);
break;
case 's': turn(1);
break;
case 'c': camera = 1;
break;
case 'v': camera = 0;
}
}
// defines timebased-actions
void idle(){
int time = glutGet(GLUT_ELAPSED_TIME);
// Alle 30 Millisekunden wird die Szene neu gezeichnet
if (time - timebase1 > 300) {
enD = tick_method();
timebase1 = time;
yx_camPos();
glutPostRedisplay();
}
if(enD == 1){
printf("Spielende, benoetigte Zuege: %d\n", whole_ticks);
exit(0);
}
}
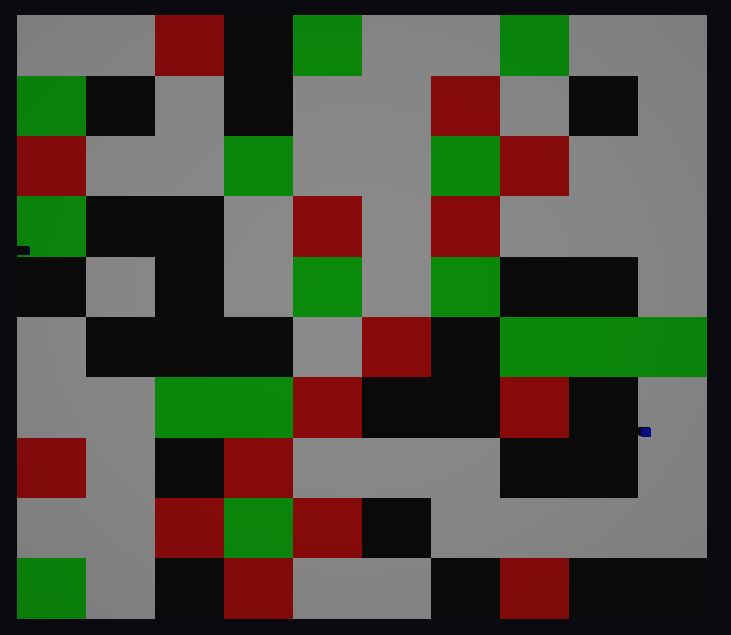